LaunchDarkly’s feature flags are a helpful tool to enable and disable application changes with a single click rather than going through an entire code deployment.
In this tutorial, we’ll use LaunchDarkly's feature flags in a JavaScript application. We’ll start with an example from my earliest days of internet building — building a fan page, which will demonstrate how feature flags work. The fan page will flip our DJ Toggle page from a 1995-style into 2000s-style site and back, depending on the state of a feature flag.
Sample Project Repository
The assets needed for this project and a completed sample are found at https://github.com/launchdarkly-labs/Risk-Management-JavaScript.
Within this repository, the assets for this tutorial can be found in the folder Feature Flag > JavaScript.
If you’re just getting started, start by creating a new directory for your project and navigating into it:
mkdir fan-page
cd fan-page
Create a LaunchDarkly Account
Head to LaunchDarkly in your web browser and create an account or log in if you already have one. If you're new, click ‘Skip setup’ after verifying your email.
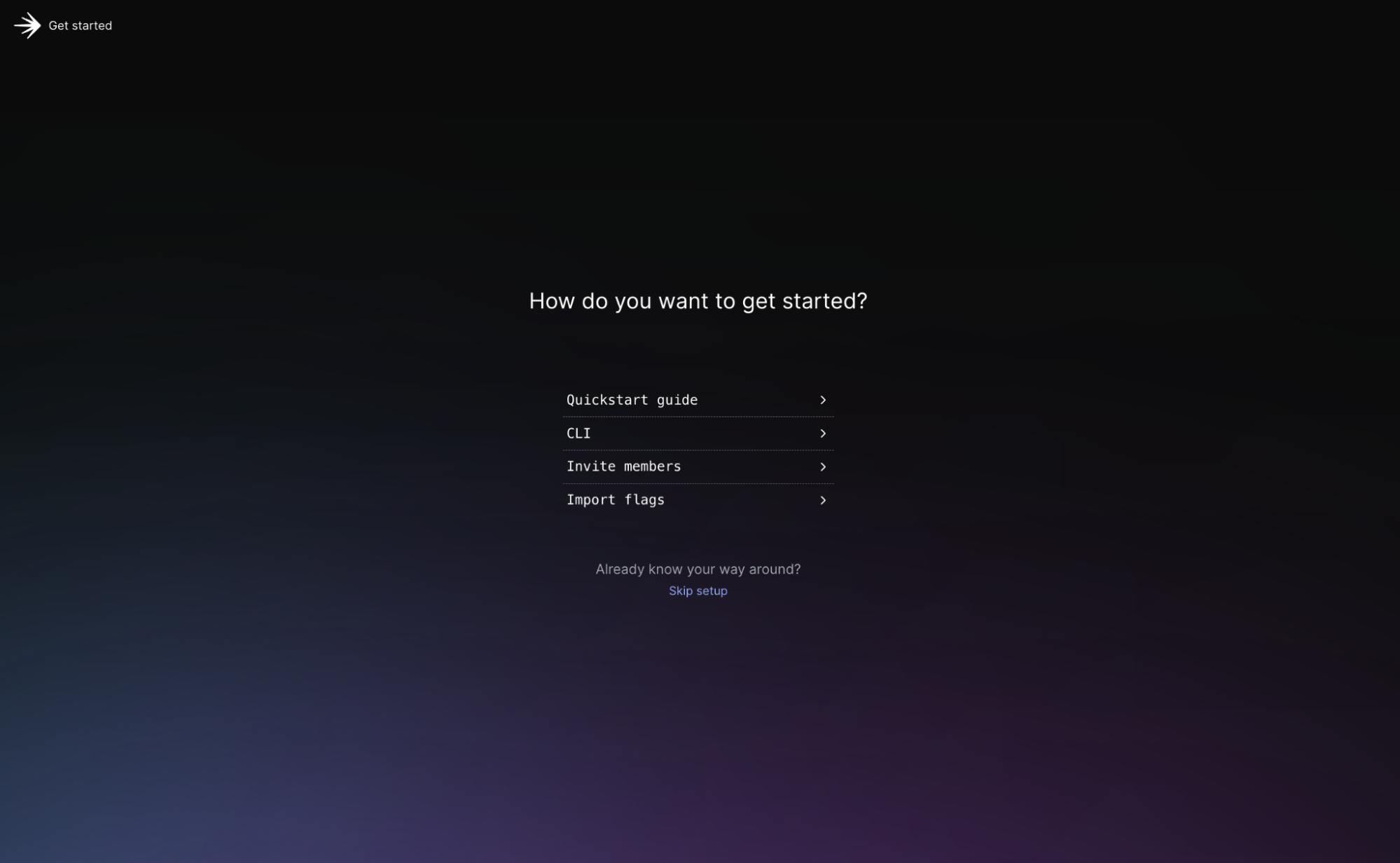
You will then be added to a default project.
To get the relevant keys for your project:
- Click on the cog icon on the lower left side of the screen.
- Navigate to "Projects" and click on your project's name.
Once you click on the project name, you’ll be brought to the project settings page.
We'll be working within our production environment. Click on the three dots next to your environment and select ‘Client-side ID’.
Create a .env file within the project folder (fan-page) by writing the following within your terminal.
echo "LD_SDK_KEY=your_actual_sdk_key_here" >> .env
Keep your secrets safe by creating a .gitignore file and adding your .env file. This will prevent any environment variables or secrets from being accidentally uploaded to GitHub.
echo ".env" >> .gitignore
Set up your JavaScript project.
Let’s set up our JavaScript project to build our fan page and update its look and feel to the 21st century.
Initialize a new NPM project in the fan-page directory:
npm init -y
This command creates a package.json file with default settings, which you'll need to manage your project dependencies.
Create a Feature Flag to switch between styles
In your new project, create a feature flag named style-update. This flag will control whether your website displays the styling of the original 1995-styled or the new-and-improved 2000s-styled webpage.
Set the flag type to Release Flag and Boolean, and check the client-side SDK checkmark. When complete, your feature flag setup should look like the below.
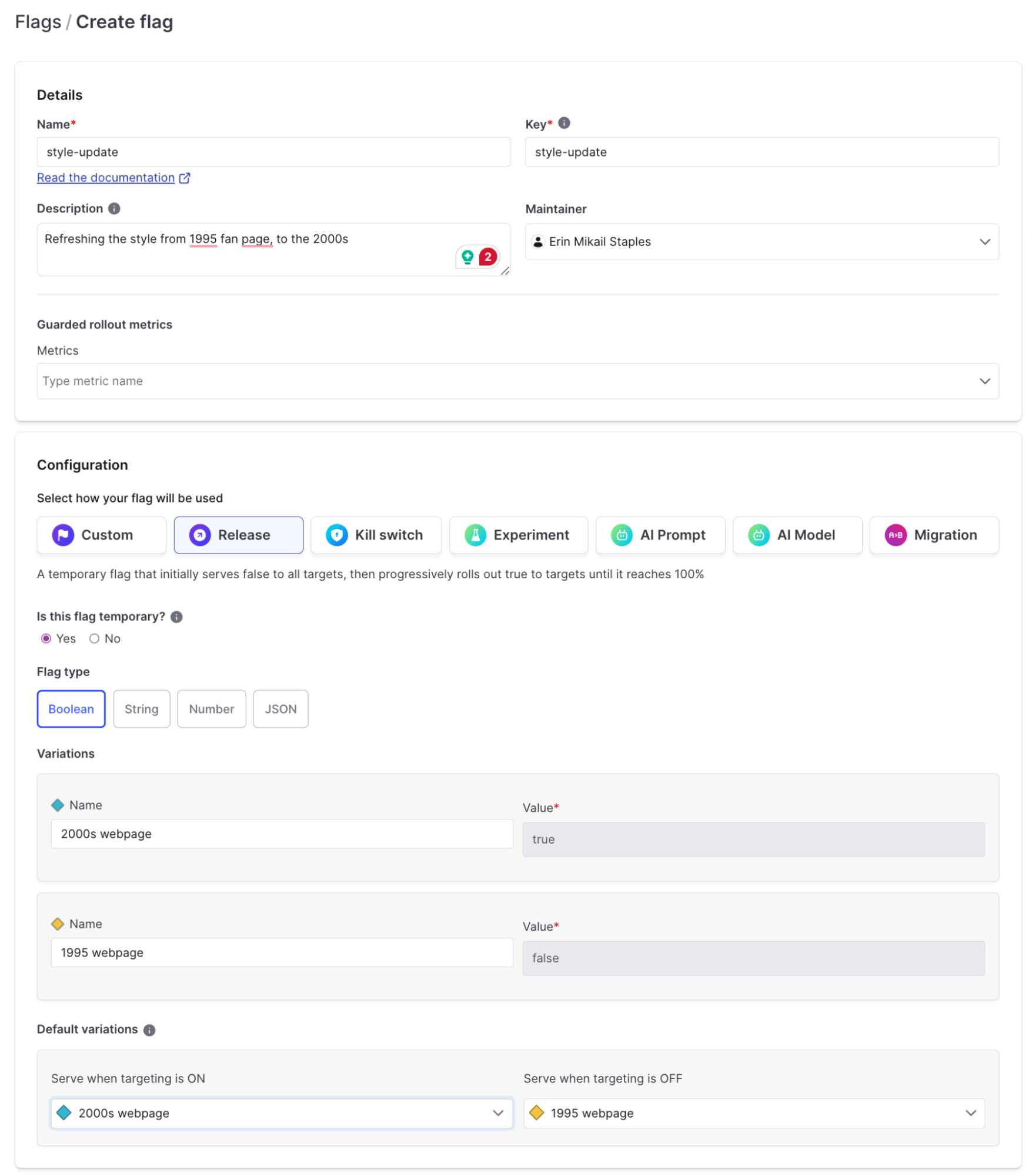
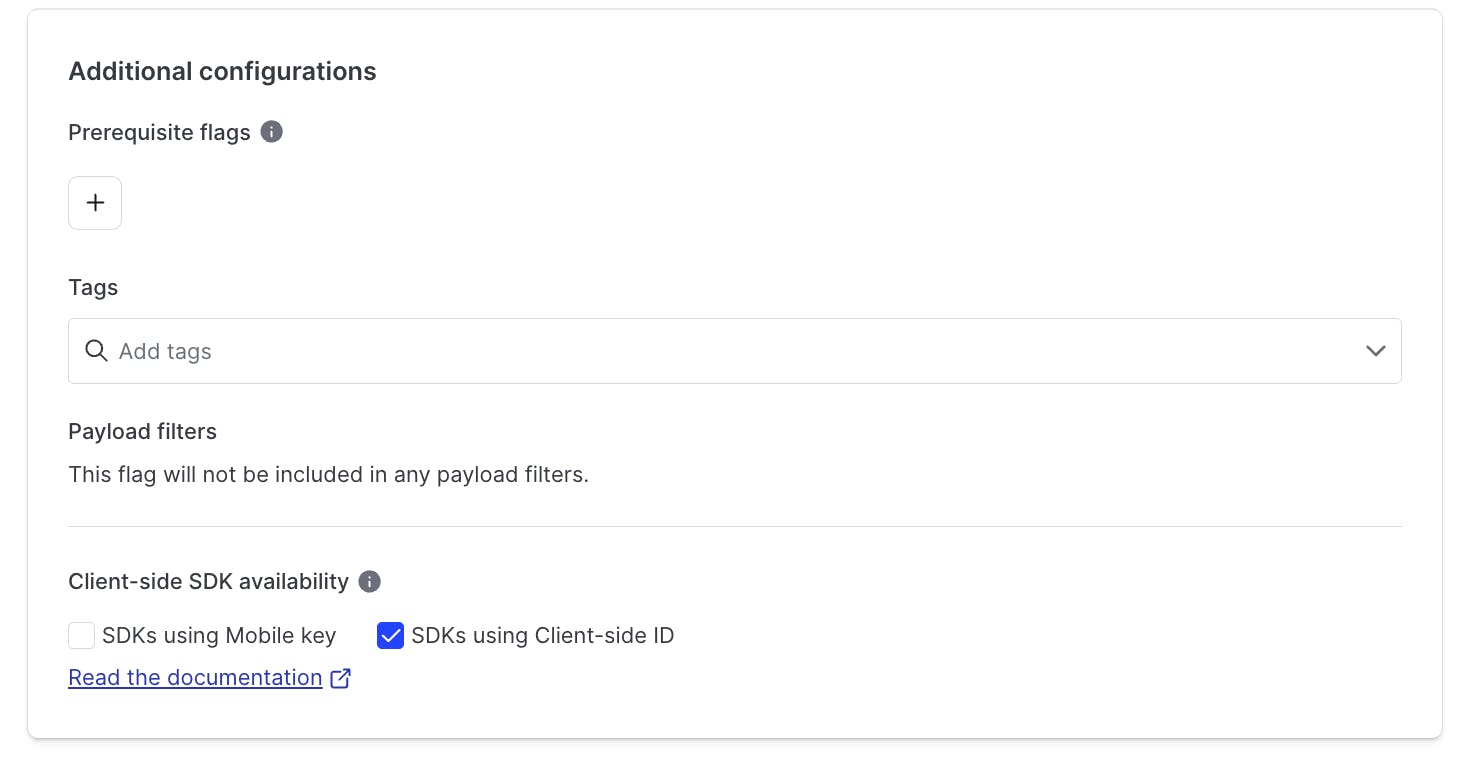
Next, install the necessary dependencies:
npm install launchdarkly-js-client-sdk dotenv-webpack
- launchdarkly-js-client-sdk: This JavaScript SDK allows your app to communicate with LaunchDarkly.
- dotenv-webpack: This package helps you manage environment variables easily.
Inside the .env file, add your LaunchDarkly Client-Side ID:
LAUNCHDARKLY_CLIENT_SIDE_ID=your_launchdarkly_client_side_id_here
Replace your_launchdarkly_client_side_id_here with the actual client-side ID from the LaunchDarkly dashboard.
Create an index.html file in your project directory.
touch index.html
Open index.html in your preferred text editor and add the following HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>DJ Toggle Fan Page</title>
<script src="https://unpkg.com/launchdarkly-js-client-sdk@2.18.1/dist/ldclient.min.js"></script>
<style id="era-styles"></style>
</head>
<body>
<div id="content"></div>
<script src="dist/bundle.js"></script>
</body>
</html>
This HTML file sets up your website's basic structure, including a div to hold the content and a style tag for custom styles that will change based on the feature flag state.
Create the JavaScript file that will control the behavior of our app based on the kill switch flag.
Create a file named app.js in the same directory:
touch app.js
Add the following JavaScript code
Open app.js in your text editor and add the following code:
const content = document.getElementById('content');
const eraStyles = document.getElementById('era-styles');
const style1995 = `
body {
background-color: #008080;
font-family: "Comic Sans MS", cursive;
color: #FFFF00;
text-align: center;
}
h1 {
font-size: 48px;
text-shadow: 2px 2px #FF00FF;
}
p {
font-size: 18px;
}
#content {
border: 5px dashed #FF00FF;
padding: 20px;
margin: 20px;
background-color: #000080;
}
`;
const style2000s = `
body {
background-color: #000000;
font-family: "Arial", sans-serif;
color: #00FF00;
text-align: center;
background-image: url('https://media.giphy.com/media/3o7aCTfyhYawdOXcFW/giphy.gif');
}
h1 {
font-size: 36px;
text-shadow: 0 0 10px #00FF00;
}
p {
font-size: 16px;
}
#content {
border: 3px solid #00FF00;
border-radius: 15px;
padding: 20px;
margin: 20px;
background-color: rgba(0, 0, 0, 0.7);
}
`;
const fanPageContent = `
<h1>Welcome to DJ Toggle's Fan Page!</h1>
<img src="https://placekitten.com/200/200" alt="DJ Toggle">
<p>DJ Toggle is LaunchDarkly's favorite DJ, known for his sick beats and feature flag flipping skills!</p>
<marquee>DJ Toggle - Flipping Flags and Dropping Beats!</marquee>
`;
const maintenanceContent = `
<h1>We'll be right back!</h1>
<p>DJ Toggle is currently fine-tuning his LaunchDarkly-powered turntables. Please check back later!</p>
`;
const ldClient = LDClient.initialize(process.env.LAUNCHDARKLY_CLIENT_SIDE_ID, {
key: 'anonymous'
});
ldClient.on('ready', function() {
const show2000sVersion = ldClient.variation('style-update', false);
if (show2000sVersion) {
content.innerHTML = fanPageContent;
eraStyles.innerHTML = style2000s;
} else {
content.innerHTML = fanPageContent;
eraStyles.innerHTML = style1995;
}
});
What does this JavaScript file do?
- Styles: We define two styles—one for a 1995 vibe with a Comic Sans font and a teal background and another for a 2000s look with a black background and Matrix-style green text.
- LaunchDarkly Integration: We initialize the LaunchDarkly client and check the value of the style-update flag. Depending on its state, we either display the 1995 version of the fan page or its new, refreshed look.
Run Your Website
To view your work in action, you’ll need to bundle your file and run it on a local server.
Run the following command to build your project using webpack:
npm run build
This command will create a dist folder with a bundle.js file containing all your JavaScript code and dependencies.
Now, serve the HTML file using a local server. We'll use the http-server package for this:
npm install -g http-server
Once installed, run the server from the root of your project directory:
http-server
By default, your files will be served at http://localhost:8080.
Opening your browser to that path, you should now see DJ Toggle's fan page in its 1995 gloriousness, yellow Comic Sans, and all.
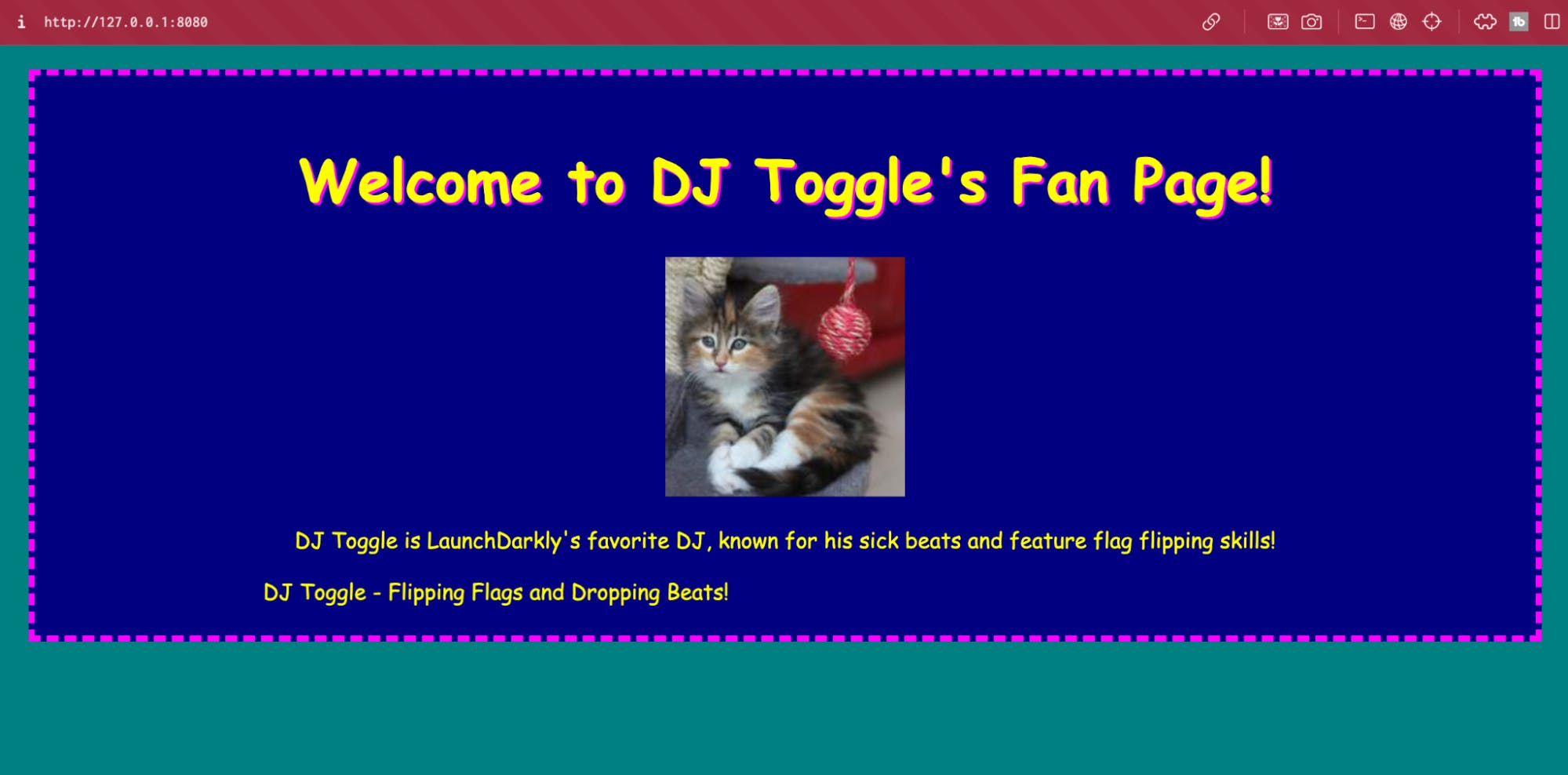
Test the Feature Flag
Now, it’s time to test out your feature flag.
Go to your LaunchDarkly dashboard and toggle the ‘style-update' flag on. Return to the website and see how its look and feel have instantly been upgraded to the early 2000s, without having to redeploy.
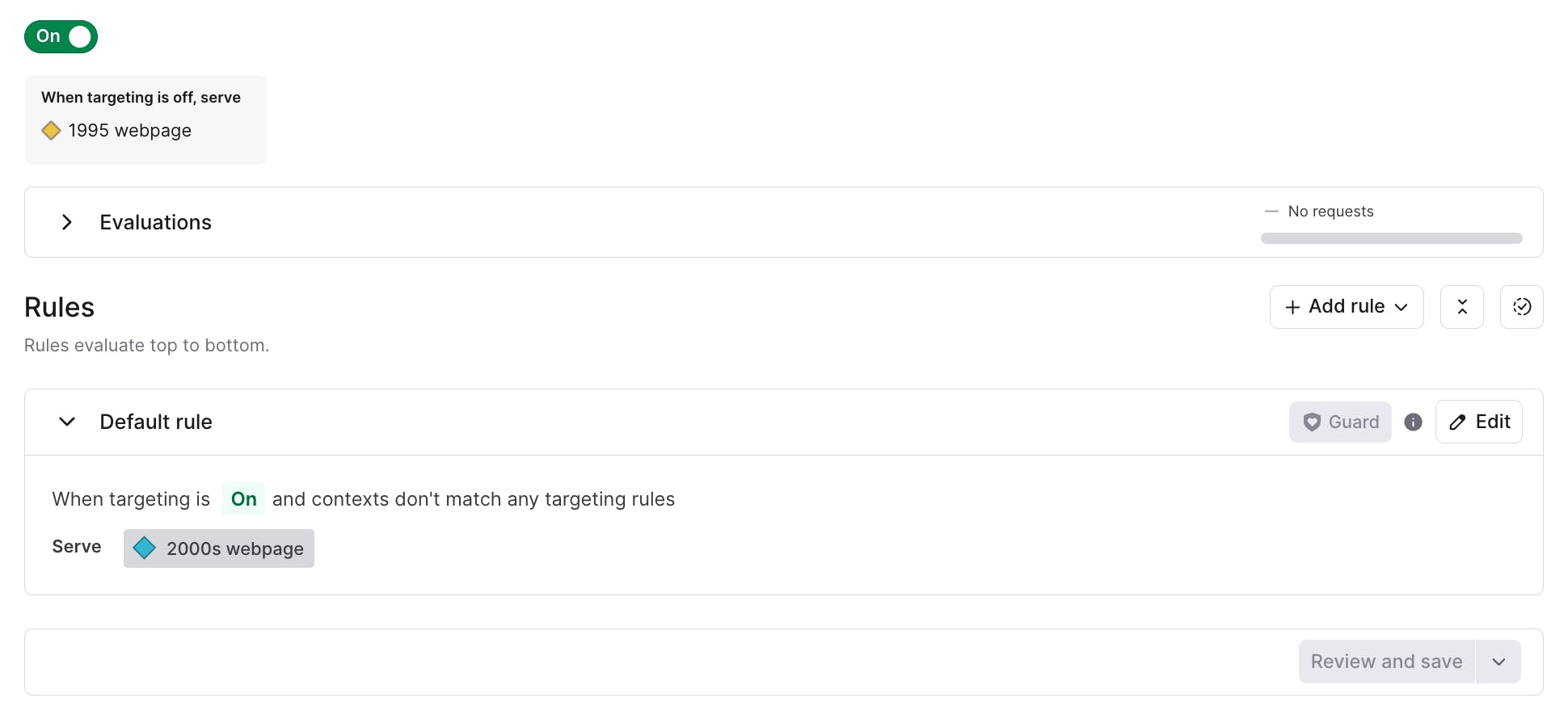
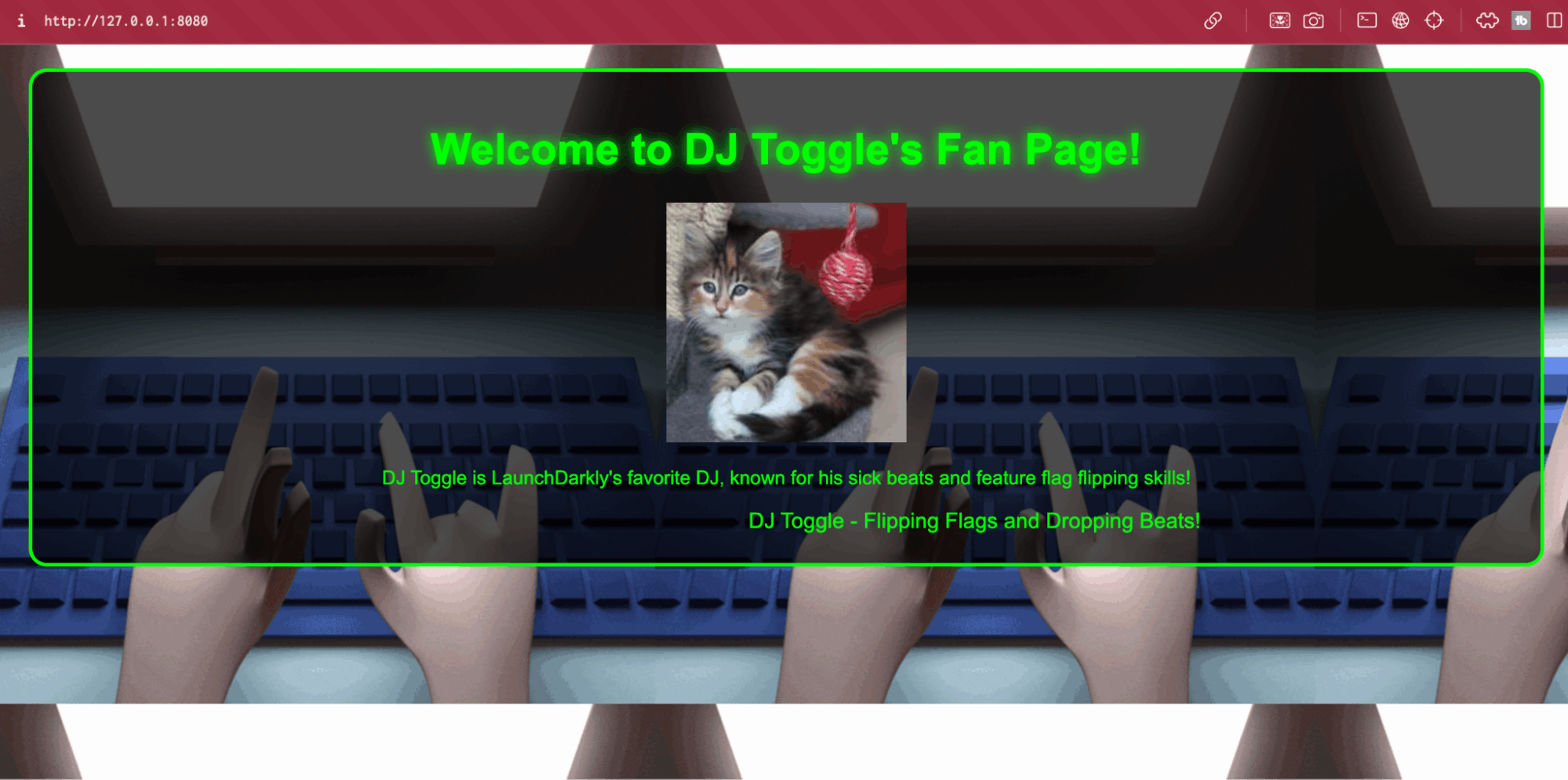
Your DJ Toggle Fan Page can instantly roll back if, heaven forbid, something were to go wrong with any feature updates that were made while updating the DJ Toggle Fan Page.
This example of using feature flags to adapt to a page’s style is just the beginning. Feature flags can be placed on different parts of your application, front or backend, allowing you to safely create your wildest dreams within your application.
When implementing new features or styles, feature flags can instantly roll back your application if something goes wrong, saving you time, money, and—let’s be honest—style points. It's like having a big, red “undo” button that you can press when things get a little too wild in production.
Conclusion
In this tutorial, you've learned how to use LaunchDarkly's feature flags to control the content and appearance of your JavaScript application. This ability to instantly roll back changes minimizes risk and keeps your users happy and your developers sane.
Check out LaunchDarkly's official documentation for more details on feature flags and other ways to mitigate risk while building to your heart's content.
Need a hand or want to geek out on how to (safely) run wild in your code? Don't hesitate to reach out— I’m always here to help. Email me at emikail@launchdarkly.com, on Twitter, LinkedIn, or join the LaunchDarkly Discord.