Feature management for JavaScript
Deploy new features faster, improve reliability, and release confidently using feature flags in JavaScript applications.
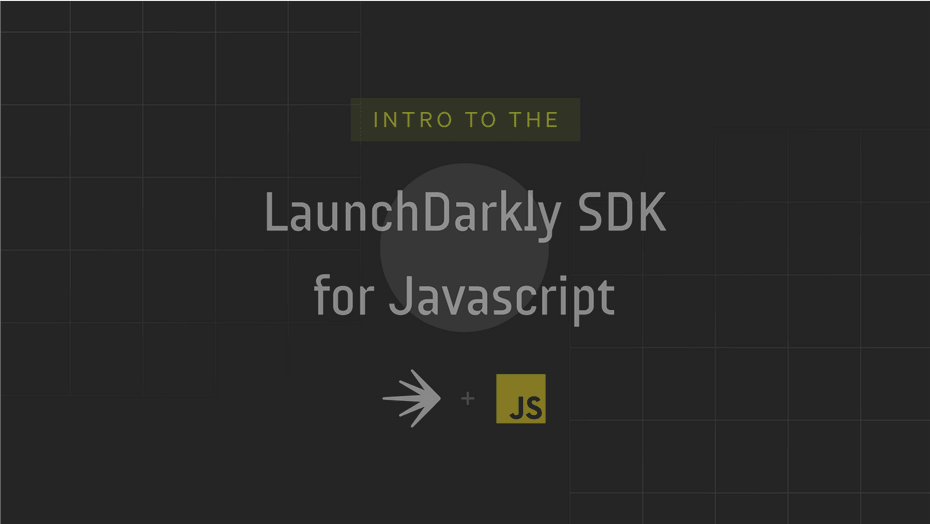
Take control of releases in JavaScript
Feature flags make it easy to deploy code changes to specific subsets of users, instantly roll back buggy features, and run A/B tests in production safely. Whether you're building with JavaScript, Node.js, React, or Vue, or developing your next desktop app in Electron, LaunchDarkly has you covered.
Small, frequent, low-risk deploys
Ship faster, confidently. LaunchDarkly feature flags decouple deployments from releases, so teams can safely test new functionality in production with the confidence to rollback instantly.
Instant rollbacks
Resolve incidents in real-time. Use feature flags like kill switches to turn off defective code with a single toggle. No emergency fixes, no redeploying an entire app.
Progressive rollouts
Use JavaScript feature flags to roll out features to a specific subset of users then gradually roll it out to the broader user base when it's ready.
Collaborative software delivery
Empower business stakeholders to turn features on and off for users. Enhance the customer experience and save engineers time. Developers can deploy code and business leaders can release features when they’re ready.
Learn more on our YouTube Channel
Watch “Static Site Generation with Next.js and LaunchDarkly”
Get started with LaunchDarkly for JavaScript
Install the SDK
// install with your favorite package manager:
npm install launchdarkly-js-client-sdk
yarn add launchdarkly-js-client-sdk
Initialize
// using ES5 require
const LDClient = require('launchdarkly-js-client-sdk');
// Using ES2015 import
import * as LDClient from 'launchdarkly-js-client-sdk';
const context = {
key: 'anonymous',
};
const client = LDClient.initialize('INSERT-YOUR-SDK-KEY', context);
Evaluate Feature Flags
client.on("ready", function () {
const showFeature = client.variation("show-feature", false);
updateFlagStatus(showFeature);
client.on("change", function (settings) {
if (settings["show-feature"]) {
updateFlagStatus(settings["show-feature"].current);
}
});
});
Feature management helps teams use feature flags on a massive scale across a variety of complex use cases.
Unlike configuration files, our feature management platform lets developers:
- Progressively roll out new features to targeted groups of users.
- Disable problematic code paths in milliseconds.
- Take complete control over every feature in a JavaScript application without having to redeploy.
Frequently Asked Questions
Nope! Our SDKs are built to be lean and have a small footprint. Check out our stats on BundlePhobia.com, https://bundlephobia.com/package/launchdarkly-js-client-sdk@3.0.0
This JavaScript SDK is for front-end client-side use. If you’re working with JavaScript on your back-end, you’ll want our Node.js server-side SDK instead: https://docs.launchdarkly.com/sdk/server-side/node-js. If you’re working with Node.js on the front-end, https://docs.launchdarkly.com/sdk/client-side/node-js
LaunchDarkly’s JavaScript SDKs all use a streaming connection, an always-open connection to the Flag Delivery Network. Thanks to this constant, high-speed connection to the flag delivery network, your connected SDKs will receive updates to feature flags within 200ms.
No, in fact, at app startup, when the SDK initializes, LaunchDarkly only needs 25ms to initialize and get the starting feature flag values from the Flag Delivery Network.
No. All our SDKs perform all feature flag evaluations in memory using the feature flag ruleset they have cached in memory.
Each of our SDKs follows the same pattern: initialize, evaluate, update. When feature flags are changed in LaunchDarkly, those changes are broadcast to the Flag Delivery Network.
LaunchDarkly reliably serves 20 trillion flags a day around the globe through our Flag Delivery Network. In the case where your app can’t reach LaunchDarkly’s flag delivery network, we’ve built in two backstops to ensure your application continues to work uninterrupted. Our SDKs are self-healing, if their connection to the flag delivery network is interrupted, they will continue to retry in the background until they can reconnect. Because all feature flag evaluations happen in memory, your app will just keep working. Each of our SDKs also offers the ability to set a default value in the event you can’t reach the flag delivery network at app startup, so your app can safely start serving your defaults and get its updates as soon as it can connect again.

We've been able to roll out new features at a pace that would've been unheard of a couple of years ago.
Discover how to deploy code faster with less risk.